Python’s for loop: usage with examples
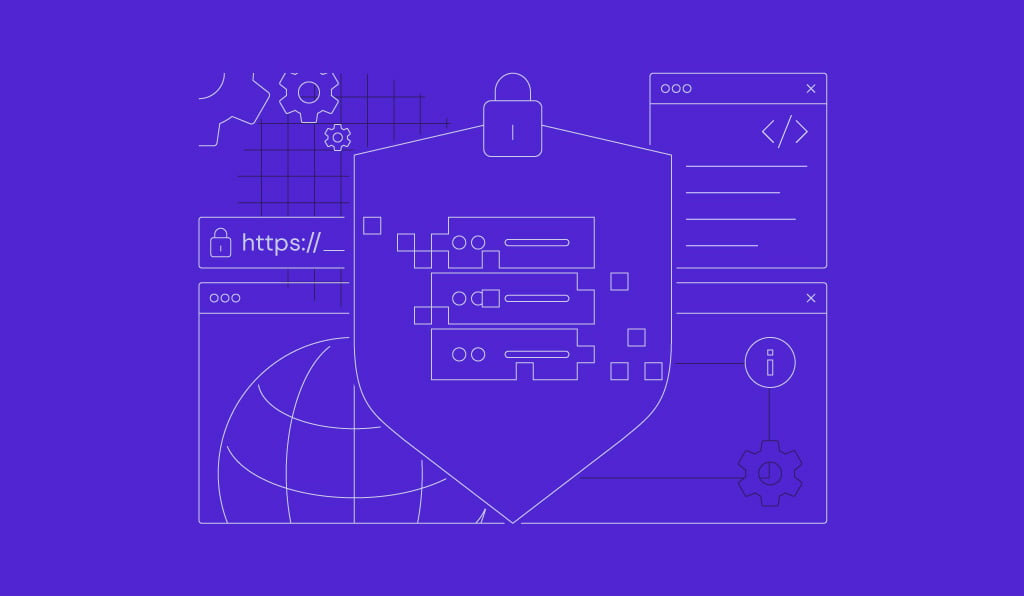
For
loop sequences are a fundamental tool in Python that enable efficient iteration, whether it’s processing data or automating repetitive tasks.
A for
loop in the Python code allows you to iterate over a sequence like a list, tuple, string, or range, and execute a block of code for each item in the sequence. It continues until there are no more elements in the sequence to process.
Imagine adding Mr. to a list of hundreds of male names. Instead of repeating the task for hundreds of times, a for
loop in Python can do this automatically. Tell the loop what list to use, and the loop picks the first item, performs the command it’s defined to do, and moves to the next item, repeating the action. This continues until all items are processed.
In this article, we’ll explain the basic syntax of a for
loop and how to use these loops in example applications. We’ll also take a closer look at how you can use Python for
loops to manage more complex tasks like server management.
Syntax of a for
loop in Python
Here’s the basic structure of the loop body:
for
loop_variable in
sequence:
Code block to execute
Component | Explanation |
for | This keyword starts the for loop. |
A loop variable | This Python loop variable takes the value of each item in the sequence, one at a time. You can name it anything you like. In our example structure, it’s loop_variable. |
in | This keyword is used to specify the sequence that you want to iterate over. |
A sequence | This is the collection of items you want to loop through. In our example structure, it’s sequence. |
Let’s say you have a list of numbers, and you want to print each number.
numbers = [1, 2, 3, 4, 5] for number in numbers: print(number)
In this loop example, the following functions and variables make this operation work:
numbers
is the sequence, which in this case is a defined list of numbers.number
is the loop variable that takes each value from the list, one at a time.print(number)
is a statement in the code block that gets executed for each item in the list.
This loop goes through each number in the list and prints it to be the following:
1 2 3 4 5
How to use a for
loop in Python
Let’s go through the mechanics of for
loops in different use cases to better understand their potential applications.
Lists and for
loops
Imagine you are managing an online store, and you want to apply a 10% discount to a list of product prices.
By iterating through the list of product prices and applying the discount, the prices can be quickly updated and displayed on the website.
# List of product prices in dollars product_prices = [100, 150, 200, 250, 300] # Discount percentage discount_percentage = 10 # Function to apply discount def apply_discount(price, discount): return price - (price * discount / 100) # List to store discounted prices discounted_prices = [] # Iterating through the list and applying the discount for price in product_prices: discounted_prices.append(apply_discount(price, discount_percentage)) # Printing the discounted prices print("Discounted Prices:", discounted_prices)
The def apply_discount(price, discount)
function takes the list of product prices and the discount percentage as arguments and returns the price after applying the discount. The discount is calculated as a percentage of the original price (price * discount / 100)
.
The for
loop iterates through each price in the product_prices
list. For each price, it runs the apply_discount
function with the current price and the discount percentage, then adds the result to the discounted_prices
list. This list is then printed as the final step of the loop.
Tuples and for
loops
Tuples are data structures that cannot be changed once defined. They are similar to lists, but are faster for processing because they cannot be modified. Tuples are written using ()
instead of the []
that lists use.
For example, let’s consider a tuple of student names and their corresponding scores. As a teacher, you need to quickly determine which students passed the exam. By iterating through the tuple of student names and scores, you can easily identify and print out the results.
# Tuple of student names and scores students_scores = (("Alice", 85), ("Lin", 76), ("Ivan", 65), ("Jabari", 90), ("Sergio", 58), ("Luisa", 94), ("Elvinas", 41)) # Passing score passing_score = 70 # Iterating through the tuple and determining who passed for student, score in students_scores: if score >= passing_score: print(f"{student} passed with a score of {score}.") else: print(f"{student} failed, scoring {score}.")
This loop example demonstrates how to use a tuple to store related data, like student names and scores. It also shows how a for
loop processes each element in the tuple, organizes it and prints the defined results.
Strings and for
loops
Strings allow you to use a for
loop to iterate over each character in the string one by one.
Imagine you’re working on a project that involves creating acronyms for various organizations. You have strings representing sentences, and you want to create an acronym from the capital letters of each word. By iterating through the string and taking the capital letter of each word, you can easily generate the desired acronyms.
def create_acronym_from_capitals(sentence): # Creating an acronym by taking only the capital letters of each word acronym = "".join(char for char in sentence if char.isupper()) return acronym # String representing a sentence sentence = "National Aeronautics and Space Administration" # Creating the acronym acronym = create_acronym_from_capitals(sentence) # Printing the acronym print(f"The acronym is: {acronym}")
Ranges and for
loops
The range()
function in Python generates a sequence of numbers. It is commonly used in for
loops to iterate over a sequence of numbers. The range()
function can take one, two, or three arguments:
range(stop)
: generates numbers from 0 to stop – 1.range(start, stop)
: generates numbers from start to stop – 1.range(start, stop, step)
: generates numbers from start to stop – 1, incrementing by step.
Suppose you’re developing an educational tool that helps students learn multiplication tables. You can use the range()
function to generate the numbers for the table. The following loop example demonstrates a solution to our example.
# Function to generate multiplication table for a given number def multiplication_table(number, up_to): for i in range(1, up_to + 1): print(f"{number} x {i} = {number * i}") # Generate multiplication table for 5 up to 10 multiplication_table(5, 10)
- Function definition: The
multiplication_table
function takes two arguments: number (thenumber
for which the table is generated) andup_to
(the range up to which the table is generated). - Using
range()
: Therange(1, up_to + 1
)generates numbers from 1 to the value ofup_to
. - Loop: The
for
loop iterates through these numbers, and for each iteration, it prints the multiplication result.
Nested for
loops
Nested loops are loops within loops. For each cycle of the outer loop, the inner loop executes every function it is defined to perform. This structure is useful for iterating over multidimensional data structures like matrices or grids.
Here’s the basic syntax for nested for
loops in Python:
for
outer_variable in
outer_sequence:
for
inner_variable in
inner_sequence:
Code to execute in the inner loop
For instance, when working on an image processing application, you need to apply a filter to each pixel in a 2D image represented as a matrix. You can use nested loops to iterate through each pixel and apply this filter.
# Example 3x3 image matrix. Here: grayscale values image_matrix = [ [100, 150, 200], [50, 100, 150], [0, 50, 100] ] # Function to apply a simple filter, for example, increasing brightness def apply_filter(value): return min(value + 50, 255) # Ensure the value does not exceed 255 # Applying the filter to each pixel for i in range(len(image_matrix)): for j in range(len(image_matrix[i])): image_matrix[i][j] = apply_filter(image_matrix[i][j]) # Printing the modified image matrix for row in image_matrix: for pixel in row: print(pixel, end=" ") print()
This loop example demonstrates how nested loops can process each element in a multidimensional data structure, which is a common requirement in image processing and other applications:
- Matrix definition. The
image_matrix
represents a 3×3 grid of pixel values. - Filter function. The
apply_filter
function increases the brightness of a pixel value, ensuring it does not exceed 255. - Nested
for
loops. The outer loop iterates through each row, and the inner loop iterates through each element in the pixel row. - Applying the filter. The filter is applied to each pixel, and the modified matrix is printed with new values.
Statements: break
and continue
with for
loops
The break
statement allows you to exit the loop before it is completed. When the break
statement is executed, the loop terminates immediately, and the program continues with the next statement following the loop.
The continue
statement allows skipping the current iteration of a loop and proceeding to the next iteration. When the continue
statement is encountered, the next iteration begins.
The break
statement
The break
statement is particularly useful when searching for an item in a list. Once the item is found, there’s no need to continue looping through the rest of the list.
In the following loop example, the loop searches for the item cherry in the list. When it finds cherry, it prints a message and exits the loop using break
. If the item is not found, the else
block is executed after the loop completes to allow for loop termination.
items = ['apple', 'banana', 'cherry', 'date', 'elderberry'] search_item = 'cherry' for item in items: if item == search_item: print(f'Found {search_item}!') break # Optional else clause else: print(f'{search_item} not found.')
The continue
statement
Consider using the continue
statement when processing a list where certain items need to be skipped based on a condition.
In the following example, the loop prints the names of fruits that do not contain the letter a. When the loop encounters an item with a in its name, the continue
statement skips the print(item)
statement for that iteration.
items = ['apple', 'banana', 'cherry', 'lemon', 'elderberry'] for item in items: if 'a' in item: continue print(item)
Function: enumerate
with for
loops
In Python, enumerate()
is a built-in function that adds a counter to an iterated item and returns it as an enumerated object. This can be especially useful for looping over a list, or any other item where both the index and the value of each item are needed.
As a developer, you can use these functions to make your code more readable and concise by eliminating the need to manually manage counter-variables. They also help reduce the risk of errors that can occur when manually incrementing a counter.
Here’s a simple example to illustrate how enumerate()
can be used in a for
loop:
# List of fruits fruits = ['apple', 'banana', 'cherry'] # Using enumerate to get index and value for index, fruit in enumerate(fruits, start=1): print(f"{index}: {fruit}")
In this loop example, enumerate(fruits, start=1)
will produce pairs of index and fruit, starting the index from 1:
1: apple 2: banana 3: cherry
Using for
loops with complex tasks
Using Python for
loops can significantly streamline the management of complex, yet repetitive processes, making routine tasks more efficient and less tedious. A great example of such a process is Virtual Private Server (VPS) management.
For
loops allow you to automate tasks like updating software packages, cleaning up log files, or restarting services. Instead of manually executing commands for each item, you can write a script that iterates over the lists you define and performs the necessary actions. This not only saves time but also ensures that your servers are consistently maintained and monitored.
Virtual servers often run on Linux-based operating systems, so make sure to catch up on installing required Python packages on Ubuntu.
You can also use VPS hosting to help with more efficient management of your own servers. Our out-of-the-box solutions are designed to simplify managing files and processes to maintain the servers. Apart from saving time, VPS hosting helps ensure the safety of your systems with built-in firewalls, SSH access, and other security measures.
Have a look at a couple of examples of how leveraging Python for
loops can make your server management workflow more efficient and less prone to errors.
Automating file processing
In the following loop example, you can use a loop to automate processing files. The following loop scans a directory, separates files from folders, and provides a list of all the files inside with their local path.
import os directory = '/path/to/directory' # Iterate over all files in the directory for filename in os.listdir(directory): file_path = os.path.join(directory, filename) if os.path.isfile(file_path): print(f'File: {filename, file_path}')
In this example, the following variables and functions make this operation work:
/path/to/directory
is a placeholder to be replaced with the local path for the directory to be processed.os.listdir(directory)
lists all the entries in the specified directory.os.path.join(directory, filename)
constructs the full path to the file.os.path.isfile(file_path)
checks if the path is a file (and not a directory).
Knowing the exact location of files helps create precise backups and restore them when needed.
Managing server logs
Here’s an example that demonstrates how to parse log files on a VPS with a Python for loop. This script reads a log file, extracts relevant information, and prints it out. For this example, we’re dealing with an Apache access log.
import re log_file_path = '/path/to/access.log' # Regular expression to parse Apache log lines log_pattern = re.compile( r'(?P<ip>\d+\.\d+\.\d+\.\d+) - - \[(?P<date>.*?)\] "(?P<request>.*?)" (?P<status>\d+) (?P<size>\d+)' ) with open(log_file_path, 'r') as log_file: for line in log_file: match = log_pattern.match(line) if match: log_data = match.groupdict() print(f"IP: {log_data['ip']}, Date: {log_data['date']}, Request: {log_data['request']}, Status: {log_data['status']}, Size: {log_data['size']}") # Example output: # IP: 192.168.1.1, Date: 01/Jan/2024:12:34:56 +0000, Request: GET /index.html HTTP/1.1, Status: 200, Size: 1024
In this example, the following variables and functions make this operation work:
- The
log_pattern
regular expression is used to match and extract parts of each log line. - The
with open(log_file_path, 'r') as log_file
statement opens the log file for reading. - The script iterates over each line in the log file, matches it against the regular expression, and extracts the relevant data.
/path/to/access.log
is a placeholder for a path to an Apache log file. A typical Apache log file consists of multiple lines with the following format and information:
192.168.1.1 - - [01/Jan/2024:12:34:56 +0000] "GET /index.html HTTP/1.1" 200 1024
The for
loop parses this information and groups it into the following categories:
IP: 192.168.1.10, Date: 01/Jan/2024:12:35:40 +0000, Request: GET /services HTTP/1.1, Status: 200, Size: 512
This script can be extended to perform various tasks, such as:
- Filtering logs based on criteria, like status codes or IP addresses.
- Generating reports on traffic, errors, or other metrics.
- Alerting on specific events, like repeated failed login attempts.
Performing system tasks
You can use a Python for
loop to automate package updates on a VPS. This script uses the subprocess module to run system commands.
import subprocess # List of packages to update packages = ["package1", "package2", "package3"] # Update package list subprocess.run(["sudo", "apt-get", "update"]) # Upgrade each package for package in packages: subprocess.run(["sudo", "apt-get", "install", "--only-upgrade", package]) print("Package updates completed.")
In this script, the package list is first updated using the sudo apt-get update
command.
Then, the script loops through each package in the packages list, and upgrades it using the sudo apt-get install --only-upgrade
command.
You need to modify the "package1", "package2", "package3"
placeholders in the packages list with the actual packages you want to update.
If you feel stuck trying to modify this script, read more about running Python scripts in Linux.
Conclusion
Mastering for
loops helps you manage Python projects more efficiently and effectively. With the for
loops, you can tackle a wide range of tasks, like automating repetitive jobs and keeping the code clean. As an example, using Python for
loops can make managing a VPS more efficient by automating updates, extracting information from logs, and generating reports based on this information.
Try to experiment with different sequences and operations to see the full potential of for
loops in action. If you have any questions or need further examples, do not hesitate to ask in the comments section.
How to write a for
loop in Python FAQ
What is a for loop in Python?
A for
loop in Python iterates over a sequence like a list, a tuple, or a string, executing a block of code for each item. It is useful for repetitive tasks, such as processing items in a list.fruits = ["apple", "banana", "cherry"]
for fruit in fruits:
print(fruit)
Can I use a for loop to iterate over a string in Python?
Yes, you can use a for
loop to iterate over a string in Python. This is useful for tasks like processing or analyzing each character in a string. In the following example, the loop iterates over each character in the string text and prints it.
How can I break out of a for loop before it completes all iterations in Python?
In Python, you can break out of a for
loop before it completes all iterations using the break
statement. This is particularly useful when you want to stop the loop after a certain condition is met, such as finding a specific item in a list.